How LimeChat builds web applications using react.
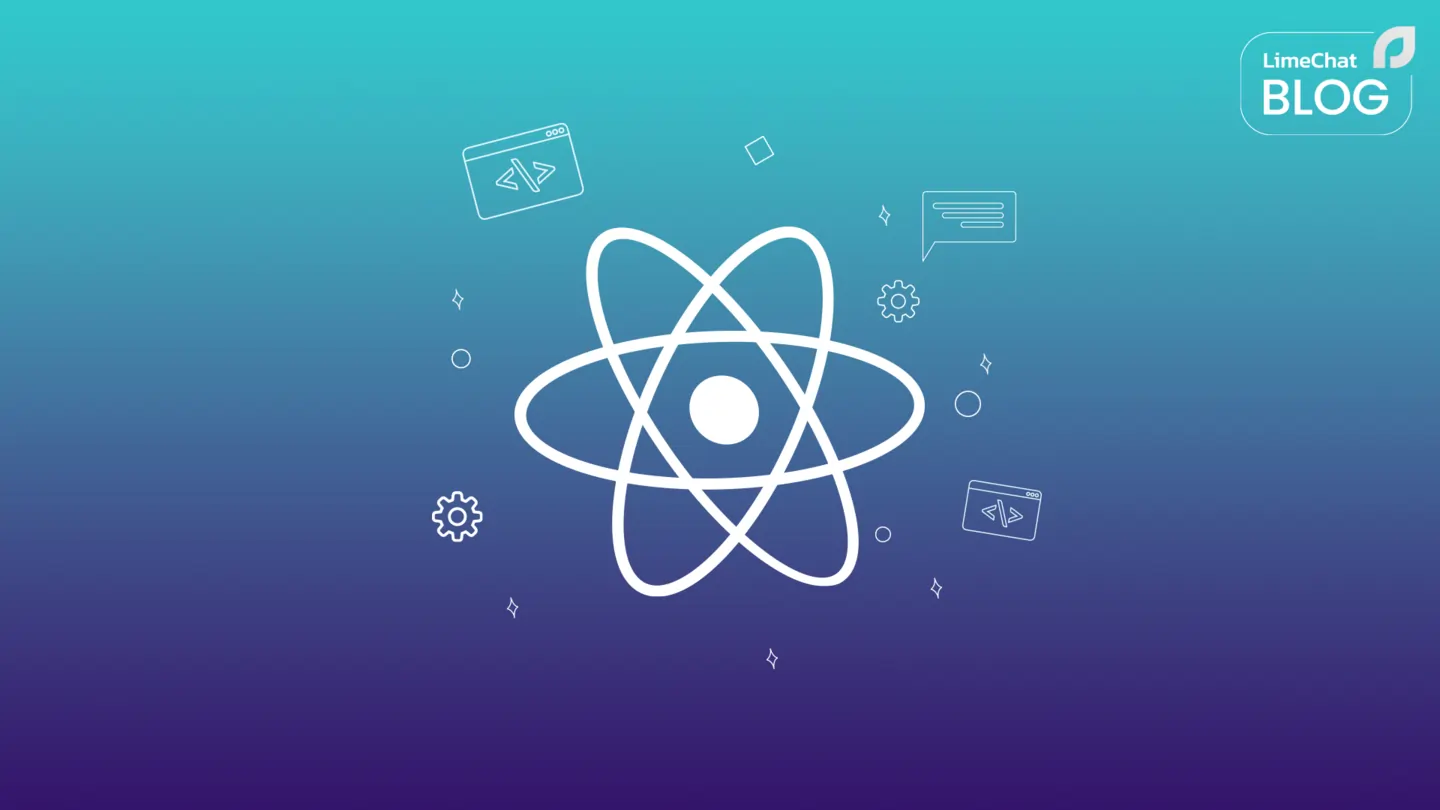
Overview
Author : Ayush Singh
It has only been a second since a new Javascript framework rolled out making it harder by day to choose the correct one for your frontend project. But even today you cannot go wrong by choosing React as your choice of weapon when it comes to building fast Single Page Application (”SPA”) from ground up in no time.
According to Stack Overflow Developer Survey 2022, React was still the 2nd most used web technology thanks to its greatly built community, plugin support and constatnt development from Meta. Here at LimeChat, we prefer it too for all for the same reasons.
Source: Stack Overflow Developer Survey 2022
Okay, but how does one go on about setting one up ready for production? That’s what we will discuss in detail in this series of blog posts. We will gover over the following points in detail and what option do we prefer here at LimeChat.
There are no correct or incorrect choices when it comes to choosing th correct tools for your React App, factor in your use case, scale, speed of development, team size etc and make decisions based on the. Because in the end it all means you do you!.
Build Tool
There are multiple choices out there when it comes to web application bundlers.
Creact React App (CRA) which is a CLI built by Facebook as the native bundler for any react application which uses Webpack in background.
**Vite** utilizes ES modules, which allows for faster builds and faster load times for the browser, as well as better support for code splitting and lazy loading.
Nx, is famous for building mono-repos 🗿which was built mainly as an Angular CLI but later extended its support to React. It provides a way to organize your code into libraries, which makes it easier to manage and reuse code across multiple applications. It even gives you option to choose between Vite and Webpack.
And many more!
At LimeChat, we use Vite 💪 as our build tool becuase as it is generally considered to be faster than Create React App (CRA) in terms of build performance and overall developer experience and has native support for react from the start.
CRA vs Vite vs NX
To get started just use on of the following commands:
And just follow the promts!
Somwhere down the promts, you will be asked to make your first decision ⚔️
Typescript vs Javascript
Both TypeScript and Babel Javascript are useful tools for modern JavaScript development, and the choice between the two depends on your specific needs and requirements. If you're looking for a more statically typed and object-oriented programming language, then TypeScript might be a better choice. If you're looking to use the latest JavaScript features in today's browsers and environments, then Babel might be a better choice. Both tools can be used together, with TypeScript being compiled to JavaScript with Babel.
At LimeChat, we generally something with faster development time and people are more well versed with Javascript hence it became the obvious choice
SWC vs Babel
You will see options for **SWC** as well, its a new Rust-based compiler with fast and efficient JavaScript compiler that can be used to compile React code, as well as other JavaScript code.
Although it may seem the best thing out there, but SWC is new at the time of writing the blog and I would choose something more well-established with a larger community and more plugins and tools available like Babel for now.
And once you choose your option, you’re done! Go inside your newly created project and Get started.
Cleanup
The boilerplate React App comes with some extra code which you won’t need so lets clean them up before moving ahead.
- Clean the react.svg from the assets folder inside the src directory, we will use this place for all kinds of assets like custom icons, font-packs, images global stylesheets etc.
- Delete the App.css , index.css and there file imports as we will setup or own stylesheets later.
Remove the React boilerplate code from App.jsx
It would look something like this after removing it.
export default App;
- Move your App.jsx and main.jsx inside src folder. (Optional, move of an individual preferance)
Setupetup your project related into in index.html
- Once done, your folder structure will look something like this now:
Styling
So React is known for its separation of concerns when it comes to JSX, so we are gonna utilise that to keep our styling separate cleaner code andeasier to edit and review our code later.
You can do this by creating module files with *.module.css files or by keeping your style separate all together in set of asset files.
Instead of choosing one, we will keep a combination of both the techniques.
Before we do that, we need to install a CSS pre-compiler like Stylus, Sass or Less (use whatever suits your need).
Global Styles
We will use Sass as an example by running the following command:
Once done, create a stylesheets folder in your assets directory and add an index.sass file inside it.
Finally, import this file inside your main.jsx
Inside this folder, you can keep all the reusable styles, styles related to external packages/plugins etc.
Modules
Keep all your component specific styles inside *.module.sass files.
For e.g.
Themeing
Themeing of your App gives it a touch unique feel according to your project and avoids repetation of code to define project colours, reusable components and font-familes etc.
Icons Library
Use any icon pack you want, we uses its homegrown icon library but you can use tabler-icons-react.
It has a great set of icons to offer, to install just do:
That’s it, you can go through its documentation to see its example use-cases.
Font Packs
Setting up custom font pack for your react project is optional, so you can skip it if your project doesn’t require one.
We will use Google’s Font Library to import font pack named Lato and set it up inside our assets directory, you can use whatever font pack as per liking.
- Inside assets folder, create a fonts directory and keep your fonts asset inside that folder.
Now create these three files _variables.sass , _mixins.sass and lato.sass .
- This is how your final folder structure will look like once you’re don.
- Now the only thing remains is to import your lato.sass inot your indes.sass file so that its Gloabally available as part of font-family.
Theme Variables
You can also create helpful variables directory according to the theme of your app. It includes your:
Component Library
Well for this particular section, yes there are multiple options out there like Material Design, AntD, Chakra, but here at limechat, we love **Mantine ❤️.**
Mantine is open source, modular anmd has a great collections of Components, Custom Hooks and much more. So can’t go wrong with this choice.
You can go to Mantine’s Get started and follow the steps for vite or whatever build tool you’re using. Or use these for now to get all mantine’s core functionality 👇
You create a customised theme which suits your app (although it would be optional).
export default theme;
Once done, add it as a top level Provider on your main.jsx
And you’re done, start using Mantine’s Components inside your code with your own cutomised theme.
This is how your final index.sass will look after all the steps.
Coding conventions
Absolute Paths
We will configure our vite to handle absolute path’s because relative paths look kinda icky 👎.
Create a jsconfig.json file to let your bundler know where the source code exists in the root directory.
Now to let vite know about this, you gotta do some changes to your vite.config.js . Before that, lets install a couple of packages we would need:
After installation, do the following changes to your vite config file to start using absolute path imports! 🥳
Linters
Setup standard linters using eslint and its AirBnB extension to make sure that the code is kept clean, we will add pre-commit on these configs as well.
Install eslint as an dev dependency and follow the promts according to your needs.
Add a .eslint.js inside the root directory to define linting rules as per your convetions.
This is what works for us 👇
Add these configs in your vite config as well
Pre-commits
To add pre-commit hooks with lint-staged, you need to follow the steps below:
nitialize husky by running the following command: npx husky-init && npm install. This will create a folder named .husky and a file named pre-commit inside it.
Replace the contents of the pre-commit file with the following command: npx lint-staged. The file should look like this:
Configure Lint Staged in your package.json file. Add the following code:
Conclusion
Now with this information, you will be able to initialize a React app with your favourite bundler, setup Styling using SasS and component libraries like Mantine and would be able to define proper linting stategies with ESLint, setup tools required for collaborative projects and basis for clean coding practices inside your project.
In the next part we will discuss further steps to setup your react application which will includes Router management, State nmanagement, Multi-Language support nad Testing and we will summarise our journey of initializing a React App.
Stay tuned for part 2! 👋
Author : Ayush Singh
Transform your marketing and support today

